- Backend Weekly
- Posts
- How Node.js Works
How Node.js Works
Learn how Node.js works behind the scene. Node.js is a powerful runtime environment built on Chrome’s V8 JavaScript engine
Hello “👋”
Welcome to another week, another opportunity to become a Great Backend Engineer.
Today’s issue is brought to you by MasteringBackend, a great resource for backend engineers. We offer next-level backend engineering training and exclusive resources.
Before we get down to the business of today. How Node.js Works.
I have a special announcement: You will love this one.
Masteringbackend is launching the beta version of its platform, which allows you to learn backend engineering in one place. The platform will help you grow your backend engineering career and turn you into a great backend engineer.
Here are some of the features:
Roadmaps => MB Roadmap enables a structured-based learning approach for Backend engineers.
Project Land => MB Projects enables backend engineers to use a learn-by-building model. Build real-world backend projects without coding the frontend.
Backend Portfolio => Create and manage your backend portfolio with many real-world backend projects.
BackLand => Learn backend engineering by solving challenges in a gamifying way.
Sound interesting?
The beta version is out for testing, reviews, and feedback.
Reply to this email if you find anything worth reporting or if there is more feedback to help us improve.
Now, back to the business of today.
I discussed Basic Terminal Commands for Backend Engineers in the previous edition. Now that we understand the basics of Backend Engineering let’s move on to Server-side languages and discuss each.
Today, I want us to look at Node.js and How it works. Let’s start with the obvious question.
What is Node.js
Node.js is a powerful runtime environment built on Chrome’s V8 JavaScript engine. It allows developers to run JavaScript code outside a web browser, enabling server-side scripting to build dynamic web applications and APIs.
From this, you can see that Node.js is not a programming language; you can compare it to being just a framework, libraries, packages, and modules built with JavaScript. However, because of the functionalities, it is preferred to be called JavaScript Runtime or JavaScript Engine.
I have been building with Node.js for the past 7+ years, yet I still ask myself this question.
Why use Node.js?
When deciding on a server-side language to use for your backend, why should you consider Node.js?
Here are a few things that I have discovered and love about Node.js and the JavaScript ecosystem.
Why Use Node.js?
Node.js offers several compelling reasons for developers to choose it for building server-side applications:
Unified Language
With Node.js, developers can use JavaScript both on the client (Frontend) and server (Backend) sides, promoting code reuse and reducing context-switching overhead.
For example, the same way you filter an array in your Frontend code is the same in your backend code, so you can reuse that piece of code.
If you filter the array like this in Frontend:
array.filter(item => item.isCompleted)
This is the same way you will do it in Node.js.
Asynchronous Programming
Node.js excels in handling I/O-intensive tasks by leveraging asynchronous programming techniques. This enables developers to write non-blocking code, improving application responsiveness and scalability.
To get more context, If you learned Java 7 like me in 2014-2015, you will notice that the language provides this Runnable interface to implement asynchronous programming or a background task.
It creates different threads and allows you to run different tasks on each thread and intercommunicate with each other.
public class ExampleClass implements Runnable {
@Override
public void run() {
System.out.println("Thread has ended");
}
public static void main(String[] args) {
ExampleClass ex = new ExampleClass();
Thread t1= new Thread(ex);
t1.start();
System.out.println("Hi");
}
}
Since Java is not an asynchronous programming language, it provides many mechanisms for asynchronous programming.
Now, imagine if you want to use threads on every executable function or method that you create. That will be a lot of threads.
In Node.js, this is done in-built. Every executable function is executed concurrently (side-by-side) without waiting for the other in Node.js, except when you explicitly indicate it.
We will discuss Asynchronous programming more as we progress.
Scalability
Node.js’s event-driven architecture and non-blocking I/O model make it well-suited for building highly scalable applications that can handle thousands of concurrent connections with minimal resource consumption.
Node.js has been battle-tested to handle millions of concurrent requests and scale efficiently.
Companies like Uber, Netflix, LinkedIn, NASA, PayPal, etc., have proven Node.js's scalability prowess beyond Doubt.
Rich Ecosystem
Node.js has a vast ecosystem of modules and packages available through npm, the Node Package Manager. Developers can easily find and integrate third-party libraries to accelerate development and add application functionality.
You can find a package or library for literally anything. For example, you can find a library for even numbers, odd numbers, etc.
npm install is-even
That is not a joke. The package has over 295K weekly downloads.

is-even NPM package
Real-time Capabilities
Node.js is ideal for building real-time applications such as chat applications, multiplayer games, and collaboration tools.
Its ability to handle concurrent connections efficiently suits scenarios requiring low-latency communication.
That’s all from me.
There are many more reasons to use Node.js. In the comment section, please tell me why you chose It.
Furthermore, let’s look at a brief history of Node.js before going deep into How Node.js Works.
History of Node.js
Node.js was born out of Ryan Dahl's vision to create a server-side platform that could leverage JavaScript's capabilities for building scalable, real-time applications.
Inception (2009): Ryan Dahl introduced Node.js to the world at the European JSConf in 2009, demonstrating its event-driven, non-blocking I/O architecture as a game-changer for server-side development.
Early Adoption: Due to its performance and simplicity, Node.js quickly gained traction among developers, particularly those working on real-time web applications and APIs.
Formation of the Node.js Foundation (2015): To foster the growth and governance of the Node.js ecosystem, the Node.js Foundation was established in 2015. It aimed to provide a neutral platform for collaboration and innovation within the community.
Release Cycle: Node.js follows a predictable release cycle, with regular releases and LTS (Long-Term Support) versions to ensure stability and security for production deployments.
Continued Evolution: The Node.js ecosystem continues to evolve, with ongoing efforts to improve performance, enhance developer experience, and expand the platform's capabilities.
That was quick.
I don’t want to spend too much time elucidating Node.js's history since you can find that with a simple Google search.
How Node.js Works
Node.js works like every other server-side programming language: you write code, compile/interpret it, and run the executable.
No.
It’s different.
The difference is what I will explore in this newsletter.
At the heart of Node.js runtime design is the Event-driven Architecture. This architecture enables the intuitive design of Node.js, making events first-class citizens.
Let’s explore it further.
Node's primary organizational principle is eliminating the blocking process through event-driven, asynchronous I/O.
Now, let’s talk about the Node Process a little:
The Node’s Process Object provides information on and controls the current running process. It is an instance of the EventEmitter, accessible from any scope, and exposes very useful low-level pointers.
If you want to learn more about Processes in Operating System, read through our previous issue on Demystifying Operating System for Backend Engineers.
Let’s look at Node Process Design.
The design shows how Node.js handles processes and uses the event-driven architecture to implement asynchronous processes.
Node Process Design
What would an environment within which many clients' jobs are cooperating scheduled look like? How are messages passed between events handled?
These questions prompt the design of the Node Process system. However, let’s examine two approaches that can solve those problems.
Collaboration
Workers can be assigned new tasks in a collaborative environment instead of idling. A virtual switchboard called Dispatcher is used.
Take a look at the illustration below:
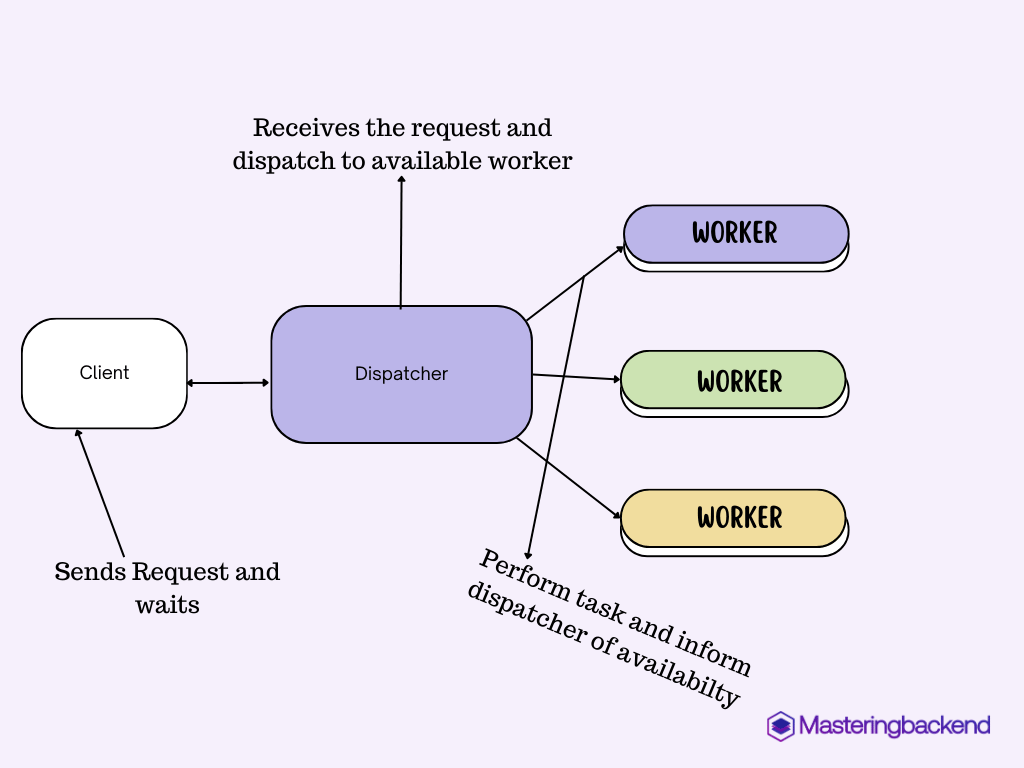
traditional Web Server Model
This approach is the traditional Web Server Model, where a web server represents the Dispatcher and distributes the job between each worker.
Queueing
A queueing approach adds a buffer (queue) between the clients and the dispatcher so as not to overwhelm anyone.
This is responsible for managing a prioritized queue of client tasks, sending and receiving requests and responses from the dispatcher and worker when idle and when not.
Node.js follows the Queueing model, and the Node system comprises 3 systems.
V8 engine (a C++ library)
Libuv (a C library)
Node (Service Manager)

Node Process Design (Event Loop)
The model is very simple:
The Node Service Manager allocates the tasks and responds to the client using a callback.
This is how it works:
All I/O operations or time-consuming operations occupy the worker pools, which the Node service manager will hand over to the dispatcher (the Libuv thread pool); otherwise, they are queued up for the event loop to pop and execute.
Every normal (non-blocking) operation is sent to the single thread of the Google V8 engine for execution.
The Node service manager runs infinitely, allocating and prioritizing tasks and collecting client responses using callback.
So it’s that simple:
The Node Service Manager is the Event Loop built into the Node.js engine to prioritize and allocate tasks to its appropriate handler.
Blocking tasks, such as working with the file system in Node.js, are handled by the Dispatcher's Libuv. The non-blocking process is processed by the Google V8 engine.
Next, we can explore Google V8 and Libuv more, learning how to build simple commands or applications with them. However, that is a story for another day.
So, if you look deeply, you will notice that Node.js is a C/C++ engine using JavaScript as a high-level syntactic sugar.
You can learn more about the Google V8 engine and use it to compile JavaScript codes. Also, you can learn more about Libuv and how to execute asynchronous I/O codes.
I hope this guide gives you a simple overview of how Node.js works behind the scenes and how a client-side language is successfully used on the server side.
That will be all for this one. See you on Saturday.
Don’t forget to Sign UP for the Beta version of Masteringbackend. It comes with unmatched benefits.
Backend Engineering Resources
Whenever you're ready
There are 4 ways I can help you become a great backend engineer:
1. The MB Platform: Join 1000+ backend engineers learning backend engineering on the MB platform. Build real-world backend projects, track your learnings and set schedules, learn from expert-vetted courses and roadmaps, and solve backend engineering tasks, exercises, and challenges.
2. The MB Academy: The “MB Academy” is a 6-month intensive Advanced Backend Engineering BootCamp to produce great backend engineers.
3. MB Video-Based Courses: Join 1000+ backend engineers learning from our meticulously crafted courses designed to empower you with the knowledge and skills you need to excel in the world of backend development.
4. MB Text-Based Courses: Access 1000+ resources, including PDF guides, comprehensive reference materials, and text-based courses that cater to learners at various stages of their backend engineering journey.
I moved my newsletter from Substack to Beehiiv, and it's been an amazing journey. Start yours here.
Join the conversation